Scalability Challenges and Solutions in React Native
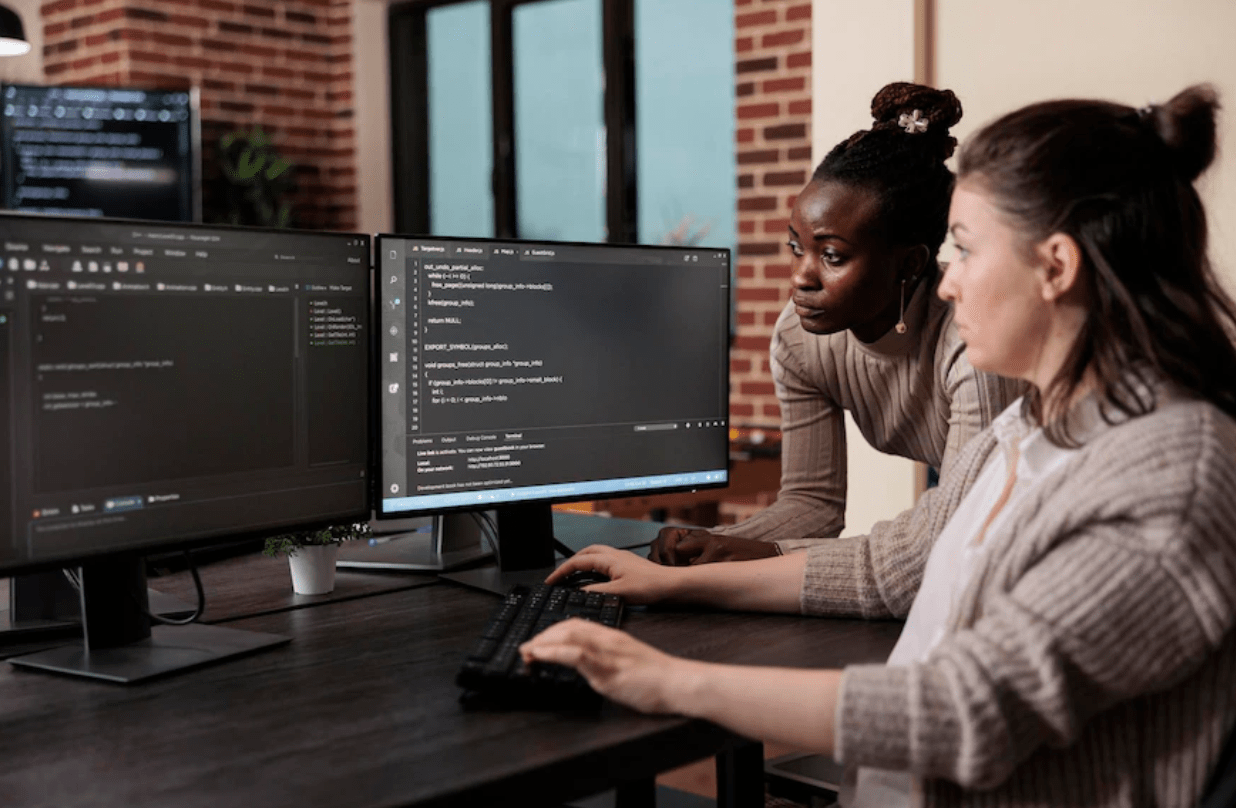
Introduction to React Native
Welcome to the world of React Native, where creating cross-platform mobile applications has become easier and faster than ever before! With its ability to write code once and deploy it on multiple platforms, React Native app development services have gained immense popularity among developers worldwide. However, as your app grows in complexity and user base, you might encounter some scalability challenges that can hinder its performance. But fear not! In this blog post, we will explore these challenges in detail and provide you with practical solutions to overcome them. So grab a cup of coffee and let’s dive into the exciting realm of scaling up your React Native apps!
Understanding Scalability Challenges in React Native
Scalability is a crucial aspect of any software development project, and React Native is no exception. While this cross-platform framework allows developers to build mobile apps using JavaScript, it does come with its own set of scalability challenges.
One challenge faced by developers is the performance impact that comes with handling large amounts of data. As the app grows and more data needs to be processed and displayed, it can lead to slower rendering times and reduced user experience. This issue becomes even more pronounced when dealing with complex UI designs or heavy animations.
Another scalability challenge in React Native involves managing state across multiple components. As an app expands in complexity, keeping track of different states across various screens can become difficult. It’s important for developers to find efficient ways to manage state changes and ensure consistency throughout the application.
Additionally, as teams grow and collaborate on larger projects, maintaining code quality becomes a challenge. With numerous developers working on different parts of the app simultaneously, it’s vital to establish coding standards and best practices from the beginning.
Furthermore, integrating third-party libraries can also present scalability challenges. While these libraries offer useful functionalities out-of-the-box, they may not always be optimized for performance or compatible with future updates.
Common Issues Faced by Developers
Developing a React Native application can be an exhilarating experience, but it does come with its fair share of challenges. As developers dive into the world of React Native, they often encounter common issues that can hinder their progress and scalability.
One of the most prevalent issues is performance optimization. Developing an app that runs smoothly on various devices and platforms requires careful attention to performance bottlenecks. It’s essential to identify and address any slow rendering or inefficient code that could impact the app’s overall speed.
Another challenge faced by developers is maintaining consistent UI across different screen sizes and resolutions. With an ever-increasing number of mobile devices available in the market, ensuring a seamless user experience becomes crucial. Adjusting layouts, typography, and images to fit different screens can be time-consuming but necessary for optimal usability.
Handling state management efficiently is also a significant concern for many developers. In complex applications with multiple components interacting with each other, managing state becomes challenging. Without proper organization and handling techniques like Redux or Context API, codebase complexity can quickly escalate.
Cross-platform compatibility is another issue plaguing developers in React Native development. While one of the primary advantages of using React Native is being able to build apps for both iOS and Android simultaneously, there are still platform-specific nuances that need addressing during development.
Debugging and troubleshooting errors effectively can also pose difficulties for developers working with React Native projects. Figuring out why something isn’t working as expected when dealing with JavaScript bridging between native modules can be quite tricky at times.
These are just some examples of the common challenges encountered by developers in their journey towards scalable React Native applications. However, armed with knowledge about these issues beforehand allows them to find suitable solutions promptly without sacrificing efficiency or quality in their work.
Techniques for Improving Scalability in React Native
When it comes to improving scalability in React Native, developers have a range of techniques at their disposal. One important technique is code-splitting, which involves dividing the app’s codebase into smaller chunks that can be loaded on-demand. This helps reduce initial load times and improve overall performance.
Another effective technique is optimizing rendering performance. By using tools like shouldComponentUpdate or PureComponent, developers can prevent unnecessary re-renders and make the app more efficient.
Caching data locally is also crucial for scalability. By implementing caching mechanisms such as AsyncStorage or Redux Persist, developers can store frequently accessed data on the device itself, reducing unnecessary network requests and improving the user experience.
Furthermore, utilizing virtualized lists is essential when dealing with large amounts of dynamic content. Libraries like FlatList or VirtualizedList help render only the items currently visible on screen, resulting in faster scrolling and smoother interactions.
Additionally, adopting a modular architecture plays a significant role in enhancing scalability. Breaking down complex components into smaller reusable ones not only makes development more manageable but also promotes code reuse and maintainability.
Employing server-side rendering (SSR) can greatly enhance scalability by offloading some processing to the server before sending it to clients. Tools like Next.js provide SSR capabilities out-of-the-box for React Native apps.
By leveraging these techniques and constantly evaluating performance bottlenecks during development cycles, you can significantly improve scalability in your React Native applications while ensuring optimal user experiences without sacrificing speed or efficiency.
Implementation of Performance Optimization Strategies
To ensure a smooth and responsive user experience, it is crucial to optimize the performance of your React Native application. Here are some strategies you can implement to enhance the overall performance of your app.
1. Code optimization: Reviewing and optimizing your codebase is essential for improving performance. Minimizing unnecessary calculations, reducing redundant code, and using efficient algorithms will contribute to faster execution times.
2. Component rendering: React Native offers various lifecycle methods that allow you to control when components update or re-render. Utilize shouldComponentUpdate and PureComponent to prevent unnecessary re-renders, improving efficiency.
3. Virtualization: Implement virtualized lists (such as FlatList or SectionList) instead of regular lists for better scrolling performance with large datasets. Virtualization only renders visible items on the screen, saving memory resources and boosting overall speed.
4. Image optimization: Images often consume significant resources in mobile applications.
Consider compressing images without compromising quality by using tools like react-native-fast-image or implementing lazy loading techniques.
5. Bundle size reduction: Keep an eye on your app’s bundle size as larger bundles take longer to download and parse.
Use tools like Webpack Bundle Analyzer or Metro Bundler’s built-in options to analyze and optimize dependencies.
6. Caching data: Store frequently accessed data locally using libraries such as AsyncStorage or RealmDB. Caching minimizes network requests, reduces latency, and improves offline functionality where applicable.
Utilizing Third-Party Libraries and Tools for Better Scalability
When it comes to improving scalability in React Native, leveraging third-party libraries and tools can be a game-changer. These external resources offer ready-made solutions that can enhance the performance and efficiency of your app development process.
One popular library is Redux, which helps manage complex state management in larger applications. By centralizing the application’s state, Redux makes it easier to scale your React Native app as it grows. It provides a predictable way of handling data flow, making debugging and testing more manageable.
Another useful tool is Expo, an open-source platform that simplifies building, deploying, and maintaining React Native apps. With Expo’s extensive range of pre-built components and native APIs, developers can save time by not having to reinvent the wheel for every feature or functionality they want to add.
For optimizing network requests and caching data efficiently, libraries like Axios or Apollo Client come in handy. These libraries provide powerful features such as request interception, response transformation, error handling mechanisms that enable efficient communication with APIs while ensuring better scalability.
Tips for Maintaining Scalability in the Long Run
1. Consistent Codebase: One of the key factors in maintaining scalability in React Native is to ensure a consistent and clean codebase. Follow best practices, adhere to coding conventions, and use modular architecture patterns like Flux or Redux to keep your code organized and maintainable.
2. Regular Refactoring: As your React Native project grows, it’s important to regularly refactor your code to eliminate any redundancies or performance bottlenecks. Refactoring not only improves readability but also enhances scalability by making it easier to add new features or make changes without impacting other parts of the application.
3. Optimize Rendering: React Native provides mechanisms like virtual lists and shouldComponentUpdate lifecycle method that can help optimize rendering performance. Utilize these techniques effectively by minimizing unnecessary re-renders and optimizing component updates.
4. Performance Testing: Perform regular performance testing on your app using tools like Reactotron or Flipper, which can provide valuable insights into potential areas of improvement. Identify any slow-performing components or inefficient algorithms and take steps to address them promptly.
5. Continuous Integration & Delivery (CI/CD): Implementing CI/CD pipelines ensures that you catch any scalability issues early on before they become major problems in production environments. Automated testing, linting, and static analysis tools can help identify potential issues at every stage of development.
6. Monitoring & Analytics: Use monitoring tools such as Sentry or Crashlytics along with analytics platforms like Google Analytics or Mixpanel to gain visibility into user behavior and monitor app performance over time. This data-driven approach allows you to proactively identify scalability issues before they impact user experience significantly.
Conclusion
Scalability is a crucial aspect of any React Native project. As your app grows in complexity and user base, it’s important to be aware of the challenges that may arise and have strategies in place to overcome them. By understanding the common issues faced by developers, implementing performance optimization techniques, utilizing third-party libraries and tools, and following best practices for maintaining scalability in the long run, you can ensure your React Native app remains robust and efficient.
Remember to regularly assess the performance of your app, identify potential bottlenecks or areas for improvement, and make necessary optimizations. Keep an eye on new updates and features provided by React Native itself as well as the community-driven ecosystem.
Scalability may seem like a daunting task at first glance but with careful planning, proper implementation of best practices, and continuous monitoring, you can tackle these challenges head-on. So go ahead and build scalable React Native apps that provide seamless experiences to users across platforms!